class PocketMon(): #상속이 목적. 객체생성 안함
def __init__(self):
self.name = ''
self.hp = 0
self.exp = 0
self.lv = 1
#상속하기 위한 메서드. 간단한 출력문만 작성. 실제 동작은 각 캐릭터의 하위 클래스에서
def 밥먹기(self):
print(self.name, '이(가) 밥을 먹는다.')
def 잠자기(self):
print(self.name, '이(가) 잠을 잔다.')
def 운동하기(self):
print(self.name, '이(가) 운동한다.')
def 놀기(self):
print(self.name, '이(가) 논다.')
def 레벨체크(self):
print(self.name, '레벨체크')
#모든 캐릭터가 동일하게 동작하므로 부모 클래스에 구현한 것을 하위에서 그대로 사용
def 상태정보(self):
print(self.name, '상태정보 출력')
print('HP:', self.hp)
print('EXP:', self.exp)
print('LV:', self.lv)
class 피카츄(PocketMon):
def __init__(self):
super().__init__()
self.name = '피카츄'
self.hp = 30 #캐릭터의 초기값 할당
def 밥먹기(self):
super().밥먹기() #부모 클래스의 밥먹기()호출
self.hp += 5
def 잠자기(self):
super().잠자기()
self.hp += 10
def 운동하기(self):
super().운동하기()
self.hp -= 10
flag = self.hp>0 #살았나 죽었나 확인한 값 flag에 저장
if flag:
self.exp += 8
self.레벨체크()
return flag
def 놀기(self):
super().놀기()
self.hp -= 5
flag = self.hp > 0
if flag:
self.exp += 3
self.레벨체크()
return flag
def 레벨체크(self):
if self.exp>=20:
self.lv += 1
print(self.name, '레벨업!')
self. exp -= 20
def 전기공격(self):
print('피카츄 백만볼트~~~~~! 피카피카!!!')
class 꼬부기(PocketMon):
def __init__(self):
super().__init__()
self.name = '꼬부기'
self.hp = 40
def 밥먹기(self):
super().밥먹기()
self.hp += 7
def 잠자기(self):
super().잠자기()
self.hp += 12
def 운동하기(self):
super().운동하기()
self.hp -= 15
flag = self.hp>0
if flag:
self.exp += 10
self.레벨체크()
return flag
def 놀기(self):
super().놀기()
self.hp -= 8
flag = self.hp > 0
if flag:
self.exp += 3
self.레벨체크()
return flag
def 레벨체크(self):
if self.exp>=25:
self.lv += 1
print(self.name, '레벨업!')
self. exp -= 25
def 물대포(self):
print('물대포 공격!! 꼬북~~!!!!')
class 파이리(PocketMon):
def __init__(self):
super().__init__()
self.name = '파이리'
self.hp = 60
def 밥먹기(self):
super().밥먹기()
self.hp += 12
def 잠자기(self):
super().잠자기()
self.hp += 22
def 운동하기(self):
super().운동하기()
self.hp -= 20
flag = self.hp>0
if flag:
self.exp += 15
self.레벨체크()
return flag
def 놀기(self):
super().놀기()
self.hp -= 10
flag = self.hp > 0
if flag:
self.exp += 7
self.레벨체크()
return flag
def 레벨체크(self):
if self.exp>=30:
self.lv += 1
print(self.name, '레벨업!')
self. exp -= 30
def 불대포(self):
print('파이리 불대포 공격!! (하지만 파이리는 말을 듣지 않았다.)')
class Menu:
def __init__(self, mon):
self.mon = mon
def run(self):
flag = True
while flag:
menu = int(input('1.밥먹기 2.잠자기 3.운동하기 4.놀기 5.상태확인 6.특기공격 7.종료'))
if menu == 1:
self.mon.밥먹기()
elif menu == 2:
self.mon.잠자기()
elif menu == 3:
flag = self.mon.운동하기()
elif menu == 4:
flag = self.mon.놀기()
elif menu == 5:
self.mon.상태정보()
elif menu == 6: #isinstance(obj, class name) obj 객체가 class name으로 만들어진 객체냐?(T/F)
if isinstance(self.mon, 피카츄): #서로 일치하지 않는 메소드를 이용하고 싶을 때
self.mon.전기공격()
elif isinstance(self.mon, 꼬부기):
self.mon.물대포()
elif isinstance(self.mon, 파이리):
self.mon.불대포()
elif menu == 7:
flag = False
if menu == '4' or menu == '3':
print('캐릭터 사망')
print('게임종료')
def main():
print('포켓몬 게임 시작')
m = input('캐릭터 선택\n1.피카츄(기본) 2.꼬부기 3.파이리')
mon = None
if m == '1':
mon = 피카츄()
if m == '2':
mon = 꼬부기()
elif m == '3':
mon = 파이리()
mon.상태정보()
mm = Menu(mon)
mm.run()
main()
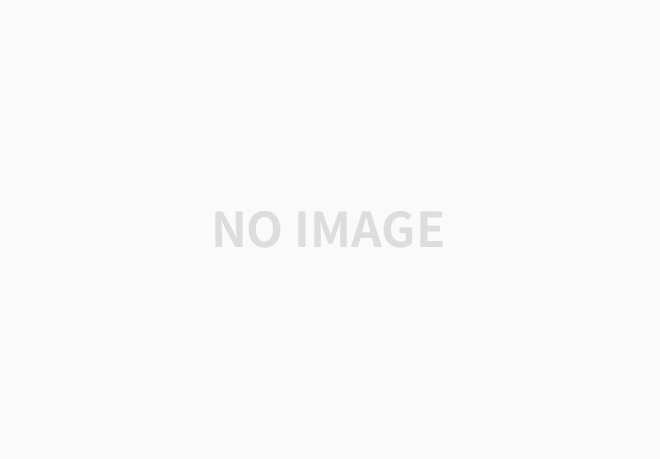
점점 class와 def를 쓰는 것이 익숙해지고 있다. 리스트나 for문을 써서 머리를 싸매는 것이 더 까탈스러움을 느낀다. 조만간 미니 팀프로젝트가 시작되는데 처음에는 무지 상태에서 프로젝트를 시작하는 것이 두려웠으나 가능성이 살짝 보인다. 한 5퍼 정도?
곧 DB가 시작되는데 괜찮겠지..?
'파이썬이 제일 쉽다면서요' 카테고리의 다른 글
python+MySQL #DB 연결 (0) | 2021.06.21 |
---|---|
python #접근제어 Private (0) | 2021.06.14 |
python #상속관계 (0) | 2021.06.14 |
python #연습문제. 제품관리 프로그램 (0) | 2021.06.13 |
python #정적멤버와 정적메소드 (0) | 2021.06.10 |